https://www.acmicpc.net/problem/2621
2621번: 카드게임
근우는 오늘 재미있는 카드 게임을 배우고 있다. 카드는 빨간색, 파란색, 노란색, 녹색의 네 가지 색이 있고, 색깔별로 1부터 9까지 숫자가 쓰여진 카드가 9장씩 있다. 카드는 모두 36(=4x9)장이다.
www.acmicpc.net
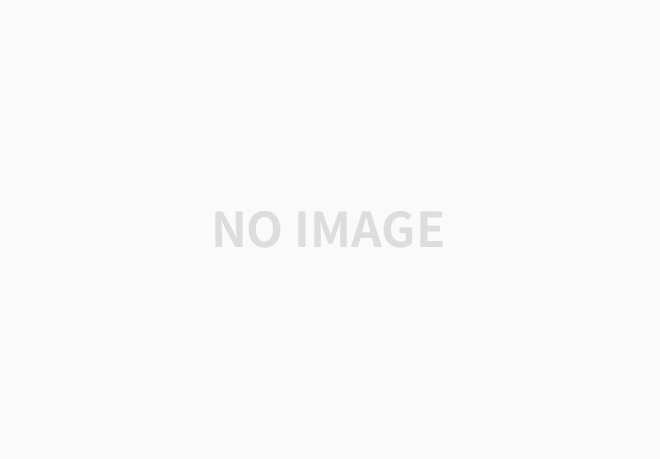
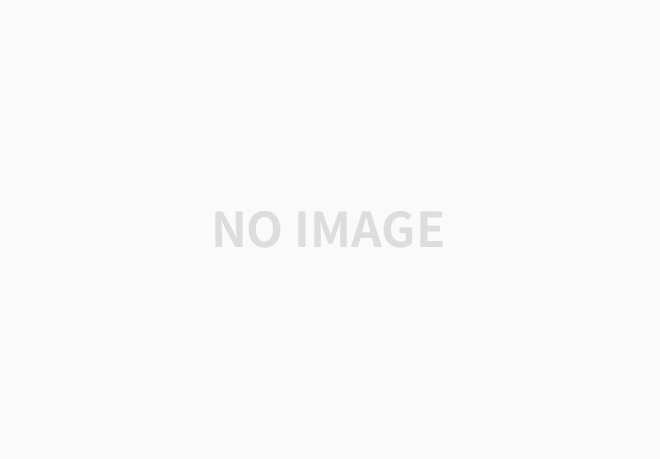
단순 구현 문제로 꼼꼼함이 가장 결국 중요한...그런 문제다.
풀면서도 규칙 9개나 되는거..너무 귀찮고...생각보다 오래걸렸다 케이스 하나하나 따지다보니
너무 귀찮아서 예제 안 돌리고 그냥 제출했더니 틀리더라
연속적 숫자인 경우가 거꾸로인 경우를 생각 못했다.
귀찮아도 예제 하나하나 입력해보는 게 결국 오류를 줄이는 것 같다.
C++은 도저히... 귀찮아서 못 하겠다.. 다음에...
파이썬 코드
#2621
import sys
input = sys.stdin.readline
cards = []
color_cnt = {
'R':0,
'B':0,
'Y':0,
'G':0,
}
number_cnt = [0]*10
def card_game():
#1 5장 모두 같은색, 숫자가 연속적.
if 5 in color_cnt.values(): #[0,5,0,0]
successive = 0
max_num = 0
for cnt, i in enumerate(number_cnt):
if number_cnt[cnt] == 1:
successive += 1
max_num = cnt
if successive == 5:
break
else:
successive = 0
continue
if successive == 5:
output = 900 + max_num
return output
#2 5장 중 4장 숫자 같음.
if 4 in number_cnt:
ind = number_cnt.index(4)
output = 800 + ind
return output
#3 5장 중 3장 숫자 같고 나머지 2장도 숫자 같음.
if 3 in number_cnt and 2 in number_cnt:
ind3 = number_cnt.index(3)
ind2 = number_cnt.index(2)
output = ind3*10 + ind2 + 700
return output
#4 5장 모두 같은 색
if 5 in color_cnt.values():
max_num=0
for cnt, i in enumerate(number_cnt):
if number_cnt[cnt] >= 1:
max_num = cnt
else:
continue
output = max_num + 600
return output
#5 5장 숫자 연속적.
successive = 0
max_num = 0
for cnt, i in enumerate(number_cnt):
if number_cnt[cnt]==1:
successive += 1
max_num = cnt
if successive == 5:
break
else:
successive = 0
continue
if successive == 5:
output = max_num + 500
return output
#6 5장 중 3장 숫자 같음.
if 3 in number_cnt:
output = number_cnt.index(3) + 400
return output
#7 5장 중 2장 숫자 같고 또 다른 2장도 숫자 같음.
twotwo=0
max_num =0
min_num =9
for cnt, i in enumerate(number_cnt):
if i == 2:
twotwo += 1
max_num = max(cnt, max_num)
min_num = min(cnt, min_num)
if twotwo == 2:
output = max_num * 10 + min_num + 300
return output
#8 5장 중 2장 숫자 같음.
if 2 in number_cnt:
output = number_cnt.index(2) + 200
return output
#9 그 외
else:
max_num = 0
for cnt, i in enumerate(number_cnt):
if i != 0:
max_num = max(max_num,cnt)
output = max_num + 100
return output
#getting ready for game
for _ in range(5):
color,num = map(str, input().split())
num = int(num)
cards.append((color, num))
color_cnt[color]+=1
number_cnt[num]+=1
cards = sorted(cards, key=lambda x: x[1])
output = card_game()
print(output)
'알고리즘 > 백준(BOJ)' 카테고리의 다른 글
[백준/BOJ/알고리즘/파이썬(python)/C++/백트래킹]#15649_N과M(1) (0) | 2021.09.06 |
---|---|
[백준/BOJ/알고리즘/DFS/그래프/파이썬(python)/C++]#2606_바이러스 (0) | 2021.09.05 |
[백준/BOJ/알고리즘/파이썬(python)/C++/그리디/정렬]#1449_수리공 항승 (0) | 2021.09.03 |
[백준/BOJ/알고리즘/파이썬(python)/C++/String]#1652_누울 자리를 찾아라 (0) | 2021.09.03 |
[백준/boj/알고리즘/파이썬(python)/C++/DFS]#11724:연결요소의 개수 (0) | 2021.09.01 |