분류(Classification)
이산적인 값 True False로 출력값을 내주는 Classification는 선형회귀의 한 타입이다.
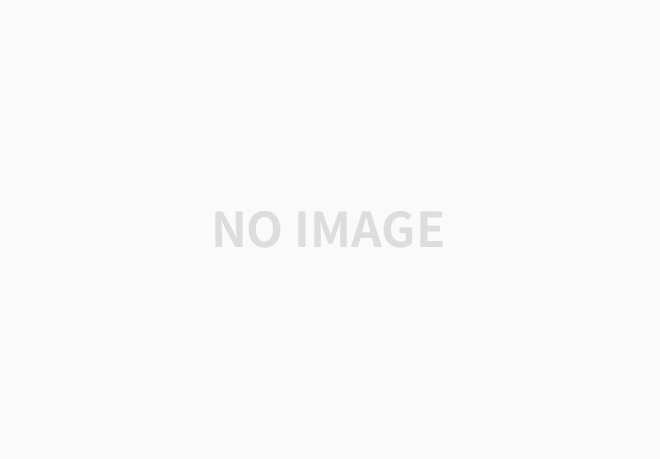
시그모이드(Sigmoid)함수
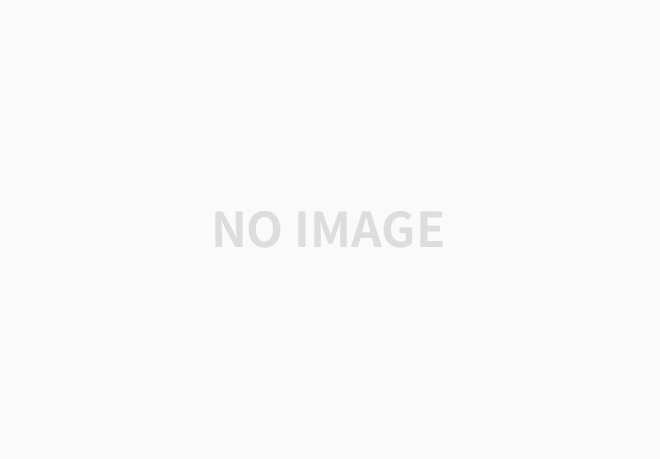
Cross-entropy
연속값을 가지던 선형회귀의 손실함수와는 다른 (이산값을 가지는 선형회귀를 위한) 손실함수가 필요하다.
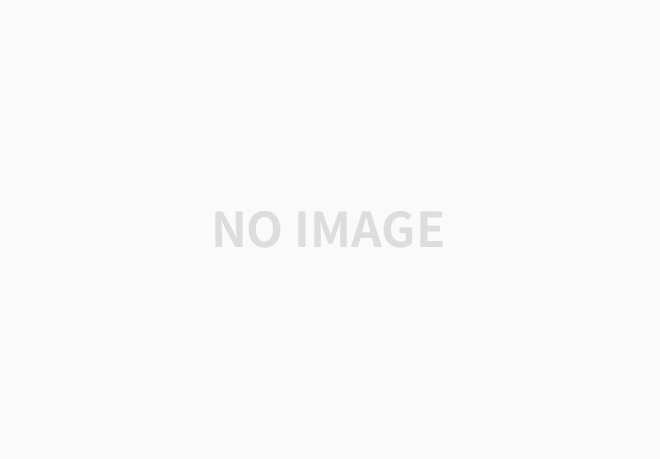
활성화 함수(Activation Function)
Sigmoid와 같이 분류작업을 하는 함수에는 RelU, Tanh도 존재한다. 머신러닝에서 세 가지 모두 잘 쓰인다.
활성화함수는 비선형함수(non-linear function)으로 입력값에 대해 비선형적 대응(분류)를 한다.
그리고 임계값(threshold)(0.5,1값 등)을 기준으로 분류를 가능하게 한다.
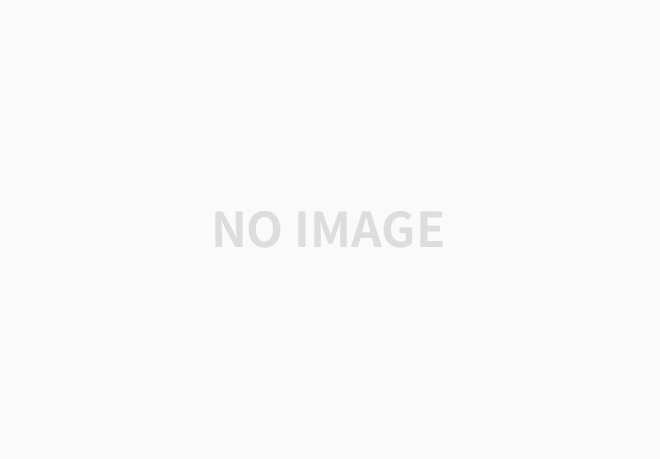
분류(Classification) 예제
예제를 통해 분류 과정을 자세히 알아보겠다.
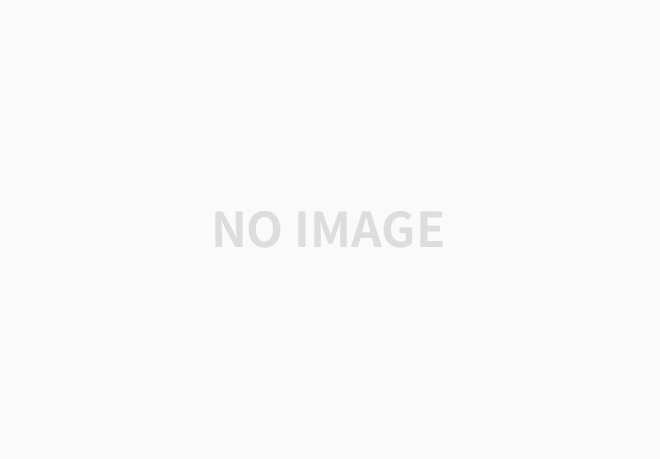
import numpy as np
#트레이닝데이터 준비
x_data=np.array([i for i in range(2,21,2)]).reshape([10,1])
y_data=np.array([0,0,0,0,0,0,1,1,1,1]).reshape([10,1])
#임의의 W,b준비
W=np.random.rand(1,1)
b=np.random.rand(1)
#Loss function
def sigmoid(x):
return 1/(1+np.exp(-x))
def loss_func(x,t):
delta=1e-7#log무한대발산 방지
z=np.dot(x,W)+b #행렬곱
y=sigmoid(z)
#-log(t*y+(t-1)*(y-1))
#cross-entropy
return -np.sum(np.log(t*(y+delta)+(t-1)*(y-1+delta)))
#수치미분
def numerical_derivative(f,input_data):
delta_x=1e-4
iter=np.nditer(input_data,flags=['multi_index'])
ret=np.zeros_like(input_data)
while not iter.finished:
idx=iter.multi_index
tmp=input_data[idx]
input_data[idx]=float(tmp)+delta_x
fx1=f(input_data)
input_data[idx]=float(tmp)+(delta_x*2)
input_data[idx]=tmp
iter.iternext()
return ret
#미래값 예측
def predict(x):
y=sigmoid(np.dot(x,W)+b)
if y<0.5:
return 0
return 1
error_val=loss_func
f=lambda x:loss_func(x_data,y_data)
learning_rate=1e-2
for step in range(8001):
W -= learning_rate*numerical_derivative(f,W)
b -= learning_rate*numerical_derivative(f,b)
if step%400==0:
print('step=',step,'error=',error_val(x_data,y_data),'W=',W,'b=',b)
print(predict(3))
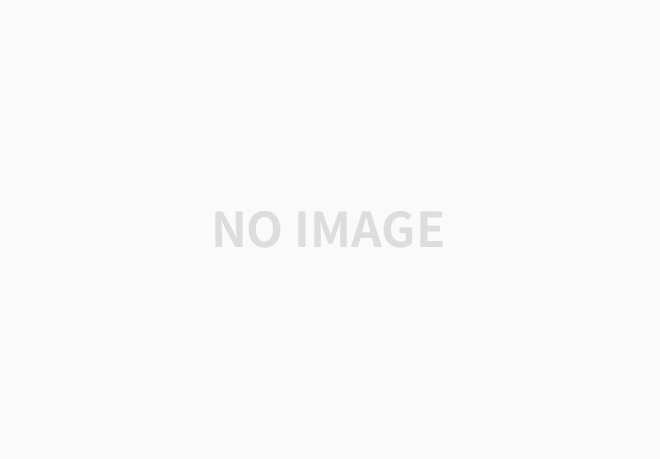
결과가 1로 True로 미래값예측이 되었다.
classification.py
0.00MB
또 다른 예제다.
예습시간과 복습시간을 입력데이터로 넣었을 경우다. 이때의 합격여부를 예측해보자.
import numpy as np
#트레이닝데이터 준비
x_data = np.array([[2, 4], [4, 11], [6, 6], [8, 5], [10, 7], [12, 16], [14, 8], [16, 3], [18, 7]]).reshape([-1, 2])
y_data = np.array([0, 0, 0, 0, 1, 1, 1, 1, 1]).reshape([-1, 1])
#임의의 W,b준비
W=np.random.rand(2,1)
b=np.random.rand(1)
#Loss function
def sigmoid(x):
return 1/(1+np.exp(-x))
def loss_func(x,t):
delta=1e-7#log무한대발산 방지
z=np.dot(x,W)+b #행렬곱
y=sigmoid(z)
#-log(t*y+(t-1)*(y-1))
#cross-entropy
return -np.sum(np.log(t*(y+delta)+(t-1)*(y-1+delta)))
#수치미분
def numerical_derivative(f,input_data):
delta_x=1e-4
iter=np.nditer(input_data,flags=['multi_index'])
ret=np.zeros_like(input_data)
while not iter.finished:
idx=iter.multi_index
tmp=input_data[idx]
input_data[idx]=float(tmp)+delta_x
fx1=f(input_data)
input_data[idx]=float(tmp)+(delta_x*2)
input_data[idx]=tmp
iter.iternext()
return ret
#미래값 예측
def predict(x):
y=sigmoid(np.dot(x,W)+b)
if y<0.5:
return 0
return 1
error_val=loss_func
f=lambda x:loss_func(x_data,y_data)
learning_rate=1e-2
for step in range(8001):
W -= learning_rate*numerical_derivative(f,W)
b -= learning_rate*numerical_derivative(f,b)
if step%400==0:
print('step=',step,'error=',error_val(x_data,y_data),'W=',W,'b=',b)
print(predict([3, 17]))
print(predict([5, 8]))
print(predict([7, 21]))
print(predict([12, 0]))
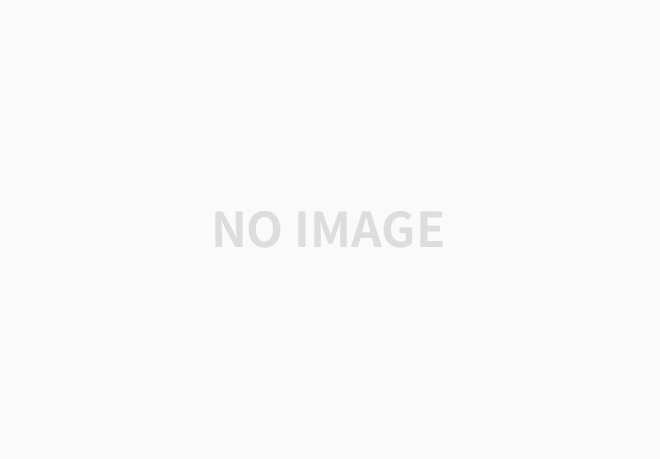
classification2.py
0.00MB
'인공지능 AI' 카테고리의 다른 글
[딥러닝/인공지능]MNIST(필기체 손글씨) (0) | 2020.06.06 |
---|---|
[딥러닝/인공지능]딥러닝기초_피드포워드/XOR문제해결 (0) | 2020.06.06 |
[머신러닝/인공지능] 선형회귀 예제 _단일변수/다변수 (0) | 2020.06.06 |
[머신러닝/인공지능] 경사하강법(Gradient Descent Algorithm) (0) | 2020.06.06 |
[머신러닝/인공지능] 수치미분(Numerical Derivative) (0) | 2020.06.06 |